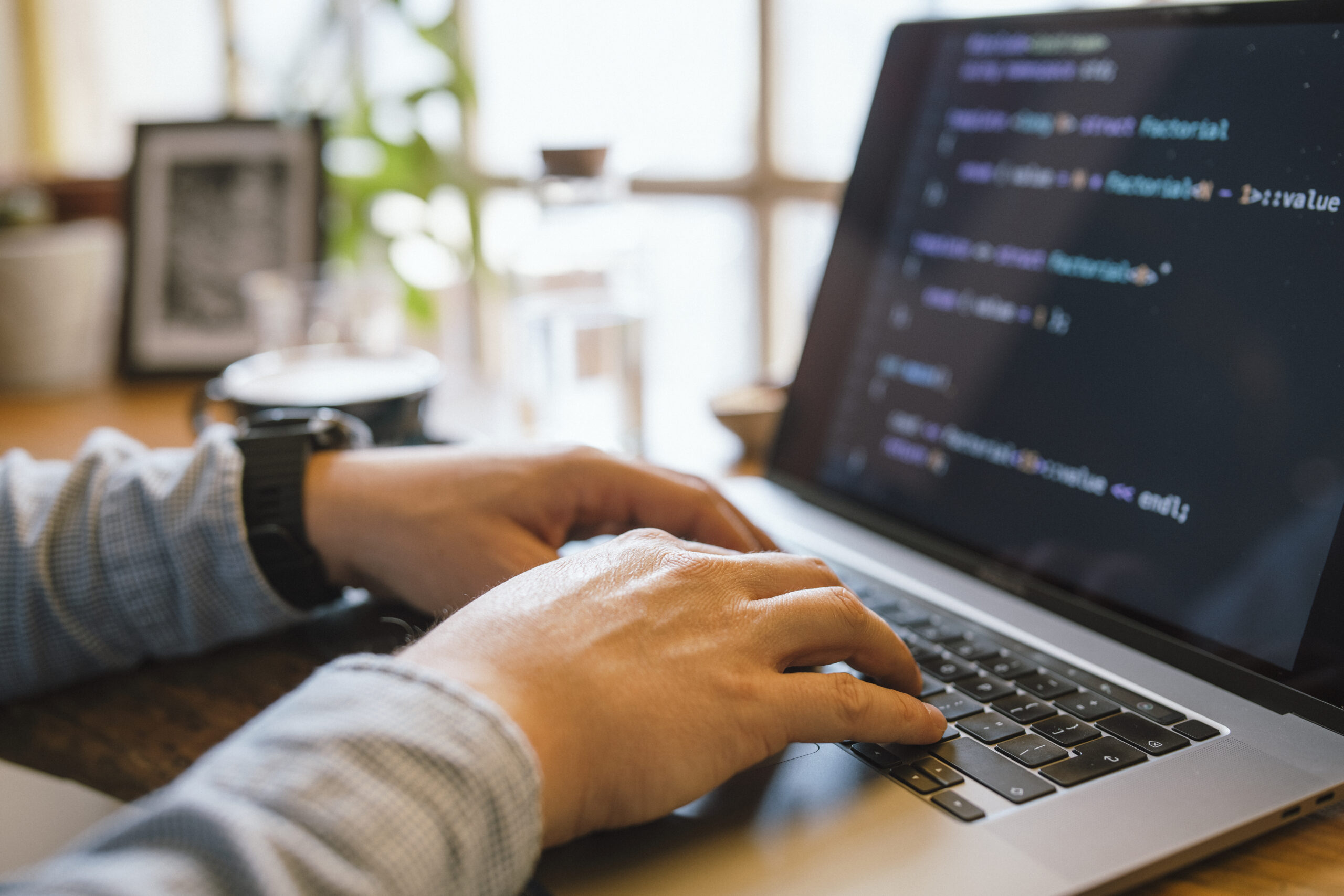
Debugging is one of the most crucial — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about comprehending how and why issues go Completely wrong, and learning to think methodically to solve problems efficiently. Regardless of whether you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and radically help your efficiency. Here's various tactics to help you developers stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest approaches builders can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is one particular Component of growth, realizing how you can connect with it properly in the course of execution is equally important. Fashionable growth environments come Geared up with effective debugging capabilities — but many builders only scratch the surface area of what these applications can do.
Take, one example is, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools enable you to set breakpoints, inspect the worth of variables at runtime, action through code line by line, and in some cases modify code within the fly. When used accurately, they let you observe accurately how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer tools, including Chrome DevTools, are indispensable for entrance-stop builders. They let you inspect the DOM, keep track of community requests, watch genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and network tabs can switch disheartening UI difficulties into manageable duties.
For backend or procedure-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory administration. Understanding these instruments might have a steeper Finding out curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with Edition Command systems like Git to comprehend code historical past, uncover the precise instant bugs had been introduced, and isolate problematic adjustments.
In the long run, mastering your instruments usually means likely beyond default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem so that when issues come up, you’re not dropped at nighttime. The higher you recognize your equipment, the more time you'll be able to shell out fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Among the most important — and sometimes disregarded — measures in efficient debugging is reproducing the issue. Before jumping into the code or earning guesses, builders want to create a consistent ecosystem or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug gets a recreation of opportunity, frequently bringing about squandered time and fragile code modifications.
The initial step in reproducing a challenge is gathering just as much context as feasible. Question concerns like: What steps resulted in the issue? Which natural environment was it in — advancement, staging, or creation? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact situations less than which the bug happens.
As you’ve collected enough data, try to recreate the problem in your neighborhood environment. This might mean inputting precisely the same data, simulating related user interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge instances or condition transitions associated. These tests not merely assistance expose the trouble and also avoid regressions Down the road.
Sometimes, The problem can be environment-certain — it would materialize only on specified functioning systems, browsers, or below unique configurations. Applying tools like virtual equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a mindset. It needs tolerance, observation, and a methodical approach. But when you can constantly recreate the bug, you happen to be by now midway to correcting it. With a reproducible scenario, You can utilize your debugging resources a lot more properly, examination likely fixes properly, and connect more Evidently with all your workforce or buyers. It turns an summary criticism right into a concrete obstacle — Which’s the place developers thrive.
Study and Comprehend the Error Messages
Error messages tend to be the most respected clues a developer has when something goes wrong. Rather then looking at them as annoying interruptions, builders need to understand to treat mistake messages as immediate communications from your method. They often tell you exactly what transpired, the place it occurred, and sometimes even why it took place — if you understand how to interpret them.
Start by studying the information meticulously and in comprehensive. A lot of developers, specially when beneath time strain, glance at the 1st line and right away start building assumptions. But deeper during the error stack or logs could lie the true root lead to. Don’t just copy and paste mistake messages into search engines like google — browse and recognize them first.
Split the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Will it level to a selected file and line selection? What module or perform induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to know the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can greatly quicken your debugging approach.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context by which the error transpired. Look at associated log entries, input values, and up to date variations in the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Discovering to interpret them properly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Properly
Logging is The most highly effective instruments in a very developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an application behaves, assisting you comprehend what’s happening under the hood without needing to pause execution or step in the code line by line.
A very good logging system begins with understanding what to log and at what level. Common logging concentrations involve DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for in depth diagnostic info during development, Facts for normal functions (like productive begin-ups), Alert for potential challenges that don’t split the appliance, Mistake for genuine troubles, and FATAL in the event the process can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure critical messages and slow down your procedure. Target important occasions, point out alterations, input/output values, and significant selection details within your code.
Structure your log messages Plainly and regularly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even simpler to parse and filter logs programmatically.
For the duration of debugging, logs let you monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments in which stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a properly-assumed-out logging method, it is possible to lessen the time it will take to spot difficulties, gain deeper visibility into your programs, and improve the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To proficiently identify and repair bugs, developers have to tactic the procedure similar to a detective solving a mystery. This state of mind can help stop working complex issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Look at the symptoms of the issue: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, gather as much appropriate facts as you may devoid of leaping to conclusions. Use logs, examination situations, and consumer reviews to piece with each other a clear image of what’s happening.
Next, variety hypotheses. Talk to you: What may very well be resulting in this habits? Have any adjustments lately been made for the codebase? Has this problem occurred right before underneath equivalent situations? The goal should be to slim down prospects and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled natural environment. In case you suspect a specific purpose or element, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the effects direct you closer to the reality.
Pay near consideration to little facts. Bugs generally conceal during the minimum envisioned areas—similar to a missing semicolon, an off-by-a person mistake, or even a race ailment. Be comprehensive and affected individual, resisting the urge to patch the issue without the need of completely being familiar with it. Short-term fixes may perhaps hide the actual issue, just for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for upcoming concerns and enable others recognize your reasoning.
By wondering like a detective, builders can sharpen their analytical competencies, strategy challenges methodically, and become more practical at uncovering concealed issues in sophisticated programs.
Generate Tests
Creating exams is among the best tips on how to improve your debugging techniques and In general development efficiency. Tests not just aid catch bugs early but in addition function a security Internet that provides you self-confidence when producing alterations to the codebase. A very well-analyzed software is simpler to debug as it lets you pinpoint particularly wherever and when a challenge occurs.
Get started with device checks, which center on particular person capabilities or modules. These smaller, isolated assessments can speedily reveal regardless of whether a particular piece of logic is Functioning as anticipated. When a test fails, you straight away know where by to glance, appreciably minimizing time spent debugging. Device assessments are Primarily handy for catching regression bugs—troubles that reappear right after previously remaining fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These help make sure a variety of areas of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that happen in elaborate programs with numerous components or expert services interacting. If one thing breaks, your checks can inform you which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To test a element correctly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way prospects to raised code structure and less bugs.
When debugging an issue, producing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails consistently, it is possible to deal with fixing the bug and observe your take a look at pass when The problem is fixed. This method makes sure that the exact same bug doesn’t return in the future.
In brief, composing checks turns debugging from the irritating guessing match right into a structured and predictable system—helping you capture additional bugs, faster and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to become immersed in the problem—looking at your display for hrs, striving Option following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
When you are much too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident problems or misreading code that you just wrote just hrs earlier. In this point out, your Mind will become fewer economical at challenge-fixing. A short wander, a espresso split, or perhaps switching to a different task for ten–15 minutes can refresh your target. Numerous builders report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also enable avert burnout, Specifically during for a longer period debugging periods. Sitting before a display screen, mentally caught, is not only unproductive and also draining. Stepping away helps you to return with renewed Electricity as well as a clearer mindset. You would possibly abruptly see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
In the event you’re trapped, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all over, stretch, or do anything unrelated to code. It may experience counterintuitive, Specifically less than tight deadlines, but it surely really brings about quicker and simpler debugging in the long run.
In a nutshell, having breaks isn't a sign of weak spot—it’s a smart method. It offers your brain Place to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of fixing it.
Master From Every Bug
Just about every bug you come upon is more than just A brief setback—It can be a possibility to develop like a developer. No matter if it’s a syntax mistake, a here logic flaw, or a deep architectural problem, each can train you a thing important if you take some time to mirror and examine what went wrong.
Begin by asking oneself several essential issues when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code opinions, or logging? The responses generally expose blind places in the workflow or being familiar with and assist you Establish much better coding behaviors transferring ahead.
Documenting bugs can be a superb behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see patterns—recurring challenges or prevalent problems—which you can proactively stay away from.
In team environments, sharing Anything you've figured out from a bug together with your friends might be Specifically potent. Whether it’s by way of a Slack message, a brief generate-up, or a quick understanding-sharing session, encouraging Some others avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. In fact, a number of the best developers are usually not the ones who generate ideal code, but individuals that constantly study from their errors.
In the long run, each bug you correct provides a fresh layer on your skill set. So upcoming time you squash a bug, take a second to replicate—you’ll come away a smarter, extra capable developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to be improved at Everything you do.